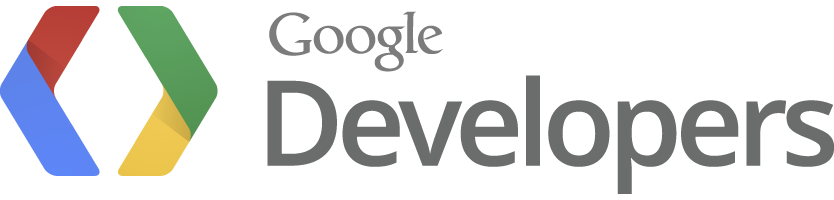
Alexander “surma” Surma
nuboLAB, GDG Berlin Golang
2013-12-12
go run -race program.go
var sharedVariable = 1 func worker() { for { sharedVariable += 1 time.Sleep(100 * time.Millisecond) } } func main() { go worker() for { fmt.Printf("> %d\n", sharedVariable) time.Sleep(1 * time.Second) } }
for {}
could starve all other goroutinesfor {}
can still starve all other goroutines. But not in realistic use-cases.cgo
you can call C code from Gocgo
now understands C++package main // int fortytwo() // { // return 42; // } import "C" import "fmt" func main() { fmt.Println(int(C.fortytwo())) // Output: 42 }
$ go test -cover *.go ok command-line-arguments 0.038s coverage: 43.2% of statementsDetails:
$ go test -coverprofile=c.out *.go $ go tool cover -func=c.out github.com/voxelbrain/auth/authenticationservice.go: init 100.0% github.com/voxelbrain/auth/authenticationservice.go: Verify 100.0% github.com/voxelbrain/auth/authenticationservice.go: verifyDataEndpoints 83.3% github.com/voxelbrain/auth/authenticationservice.go: verifyAuthenticationEndpoints 82.4% github.com/voxelbrain/auth/authenticationservice.go: AuthenticationServiceHandler 0.0% // ... total: (statements) 43.2%
$ go tool cover -html=c.out -o coverage.html
type BinaryFunc(a, b int) int var f BinaryFuncThe old way:
f = func(a, b int) int { return someStruct.BinaryMethod(a, b) }Now:
f = someStruct.BinaryMethod
text/template
now has eq, ne, lt, gt, …
fmt.Sprintf("%[3]c %[1]c %c\n", 'a', 'b', 'c')
os/fsnotify
cgo
: Objective-C supportQuestions?
emaila.surma@nubolab.org
g++AlexanderSurma
twittersurmair
githubsurma